Rust 是什么
Rust 是一门赋予每个人构建可靠且高效软件能力的语言。
-
高性能:速度惊人且内存利用率极高
-
可靠性:在编译期就能消除各种内存错误
-
生产力:出色的文档,友好的编译器和清晰的错误提示信息
为什么要用 Rust 进行嵌入式开发
Rust 的设计理念:既要安全,也要高性能。Rust 的设计理念完全是嵌入式开发所需要的。
嵌入式软件在运行过程中出现问题,大部分是由于内存引起的。Rust 语言可以说是一门面向编译器的语言。在编译期间,就能够确保你安全地使用内存。
目前,嵌入式的主流开发语言还是 C 语言,不能上来就把底层的逻辑用 Rust 重新实现一遍。但是可以在 C 代码中嵌入 Rust 语言。
C 调用 Rust
在 C 代码中调用 Rust 代码,需要我们将 Rust 源代码打包为静态库文件。在 C 代码编译时,链接进去。
创建 lib 库
1、在 Clion 中使用cargo init --lib rust_to_c
建立 lib 库。添加以下代码到 lib.rs 中,使用 Rust 语言计算两个整数的和:
1#![no_std]
2usecore::PanicInfo;
3
4#[no_mangle]
5pubextern"C"fnsum(a:i32,b:i32)->i32{
6a+b
7}
8
9#[panic_handler]
10fnpanic(_info:&PanicInfo)->!{
11loop{}
12}
在 Cargo.toml 文件中添加以下代码,生成静态库文件:
1[lib]
2name="sum"
3crate-type=["staticlib"]
4path="src/lib.rs"
交叉编译
1、安装 armv7 target:
1rustuptargetaddarmv7a-none-eabi
2、生成静态库文件:
1PSC:UsersLiuKangDesktopRUST
ust_to_c>cargobuild--target=armv7a-none-eabi--release--verbose
2Freshrust_to_cv0.1.0(C:UsersLiuKangDesktopRUST
ust_to_c)
3Finishedrelease[optimized]target(s)in0.01s
生成头文件
1、安装cbindgen](https://github.com/eqrion/cbindgen)),cbindgen从 rust 库生成 C/C++ 11 头文件:
1cargoinstall--forcecbindgen
2、在项目文件夹下新建文件cbindgen.toml
文件:
3、生成头文件:
1cbindgen--configcbindgen.toml--craterust_to_c--outputsum.h
调用 Rust 库文件
1、将生成的sum.h
以及sum.a
文件放入rt-threadspqemu-vexpress-a9applications
目录下
2、修改 SConscript 文件,添加静态库:
1frombuildingimport*
2
3cwd=GetCurrentDir()
4src=Glob('*.c')+Glob('*.cpp')
5CPPPATH=[cwd]
6
7LIBS=["libsum.a"]
8LIBPATH=[GetCurrentDir()]
9
10group=DefineGroup('Applications',src,depend=[''],CPPPATH=CPPPATH,LIBS=LIBS,LIBPATH=LIBPATH)
11
12Return('group')
3、在 main 函数中调用 sum 函数, 并获取返回值
1#include
2#include
3#include
4#include
5#include"sum.h"
6
7intmain(void)
8{
9int32_ttmp;
10
11tmp=sum(1,2);
12printf("callrustsum(1,2)=%d
",tmp);
13
14return0;
15}
4、在 env 环境下,使用 scons 编译工程:
1LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
2$scons-j6
3scons:ReadingSConscriptfiles...
4scons:donereadingSConscriptfiles.
5
6scons:warning:youdonotseemtohavethepywin32extensionsinstalled;
7parallel(-j)buildsmaynotworkreliablywithopenPythonfiles.
8File"D:softwareenv_released_1.2.0env oolsPython27Scriptsscons.py",line204,in<module>
9scons:Buildingtargets...
10scons:buildingassociatedVariantDirtargets:build
11LINKrtthread.elf
12arm-none-eabi-objcopy-Obinaryrtthread.elfrtthread.bin
13arm-none-eabi-sizertthread.elf
14textdatabssdechexfilename
15628220214886700717068af10crtthread.elf
16scons:donebuildingtargets.
17
18LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
19$qemu.bat
20WARNING:Imageformatwasnotspecifiedfor'sd.bin'andprobingguessedraw.
21Automaticallydetectingtheformatisdangerousforrawimages,writeoperationsonblock0willberestricted.
22Specifythe'raw'formatexplicitlytoremovetherestrictions.
23
24|/
25-RT-ThreadOperatingSystem
26/|4.0.4buildJul282021
272006-2021Copyrightbyrt-threadteam
28lwIP-2.1.2initialized!
29[I/sal.skt]SocketAbstractionLayerinitializesuccess.
30[I/SDIO]SDcardcapacity65536KB.
31[I/SDIO]switchingcardtohighspeedfailed!
32callrustsum(1,2)=3
33msh/>
加减乘除
1、在 lib.rs 文件中,使用 rust 语言实现加减乘除运算:
1#![no_std]
2usecore::PanicInfo;
3
4
5#[no_mangle]
6pubextern"C"fnadd(a:i32,b:i32)->i32{
7a+b
8}
9
10#[no_mangle]
11pubextern"C"fnsubtract(a:i32,b:i32)->i32{
12a-b
13}
14
15#[no_mangle]
16pubextern"C"fnmultiply(a:i32,b:i32)->i32{
17a*b
18}
19
20#[no_mangle]
21pubextern"C"fndivide(a:i32,b:i32)->i32{
22a/b
23}
24
25#[panic_handler]
26fnpanic(_info:&PanicInfo)->!{
27loop{}
28}
2、生成库文件和头文件并放在 application 目录下
3、使用 scons 编译,链接时报错,在 rust github 仓库的 issues 中找到了解决办法(https://github.com/rust-lang/compiler-builtins/issues/353):
1LINKrtthread.elf
2d:/software/env_released_1.2.0/env/tools/gnu_gcc/arm_gcc/mingw/bin/../lib/gcc/arm-none-eabi/5.4.1/armv7-ar/thumblibgcc.a(_arm_addsubdf3.o):Infunction`__aeabi_ul2d':
3(.text+0x304):multipledefinitionof`__aeabi_ul2d'
4applicationslibsum.a(compiler_builtins-9b744f6fddf5e719.compiler_builtins.20m0qzjq-cgu.117.rcgu.o):/cargo/registry/src/github.com-1ecc6299db9ec823/compiler_builtins-0.1.35/src/float/conv.rs:143:firstdefinedhere
5collect2.exe:error:ldreturned1exitstatus
6scons:***[rtthread.elf]Error1
7scons:buildingterminatedbecauseoferrors.
4、修改rtconfig.py
文件, 添加链接参数--allow-multiple-definition
:
1DEVICE='-march=armv7-a-marm-msoft-float'
2CFLAGS=DEVICE+'-Wall'
3AFLAGS='-c'+DEVICE+'-xassembler-with-cpp-D__ASSEMBLY__-I.'
4LINK_SCRIPT='link.lds'
5LFLAGS=DEVICE+'-nostartfiles-Wl,--gc-sections,-Map=rtthread.map,-cref,-u,system_vectors,--allow-multiple-definition'+
6'-T%s'%LINK_SCRIPT
7
8CPATH=''
9LPATH=''
5、编译并运行 qemu:
1LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
2$scons-j6
3scons:ReadingSConscriptfiles...
4scons:donereadingSConscriptfiles.
5
6scons:warning:youdonotseemtohavethepywin32extensionsinstalled;
7parallel(-j)buildsmaynotworkreliablywithopenPythonfiles.
8File"D:softwareenv_released_1.2.0env oolsPython27Scriptsscons.py",line204,in<module>
9scons:Buildingtargets...
10scons:buildingassociatedVariantDirtargets:build
11LINKrtthread.elf
12arm-none-eabi-objcopy-Obinaryrtthread.elfrtthread.bin
13arm-none-eabi-sizertthread.elf
14textdatabssdechexfilename
15628756214886700717604af324rtthread.elf
16scons:donebuildingtargets.
17
18LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
19$qemu.bat
20WARNING:Imageformatwasnotspecifiedfor'sd.bin'andprobingguessedraw.
21Automaticallydetectingtheformatisdangerousforrawimages,writeoperationsonblock0willberestricted.
22Specifythe'raw'formatexplicitlytoremovetherestrictions.
23
24|/
25-RT-ThreadOperatingSystem
26/|4.0.4buildJul282021
272006-2021Copyrightbyrt-threadteam
28lwIP-2.1.2initialized!
29[I/sal.skt]SocketAbstractionLayerinitializesuccess.
30[I/SDIO]SDcardcapacity65536KB.
31[I/SDIO]switchingcardtohighspeedfailed!
32callrustsum(1,2)=3
33callrustsubtract(2,1)=1
34callrustmultiply(2,2)=4
35callrustdivide(4,2)=2
Rust 调用 C
可以 在 C 代码中调用 Rust,那么在 Rust 中也可以调用 C 代码。我们在 Rust 代码中调用 rt_kprintf 函数:
修改 lib.rs 文件
1//导入的rt-thread函数列表
2extern"C"{
3pubfnrt_kprintf(format:*constu8,...);
4}
5
6#[no_mangle]
7pubextern"C"fnadd(a:i32,b:i32)->i32{
8unsafe{
9rt_kprintf(b"thisisfromrust
"as*constu8);
10}
11a+b
12}
生成库文件
1cargobuild--target=armv7a-none-eabi--release--verbose
2Compilingrust_to_cv0.1.0(C:UsersLiuKangDesktopRUST
ust_to_c)
3Running`rustc--crate-namesum--edition=2018src/lib.rs--error-format=json--json=diagnostic-rendered-ansi--crate-typestaticlib--emit=dep-info,link-Copt-level=3-Cembed-bitcode=no-Cmetadata=a
40723fa112c78339-Cextra-filename=-a0723fa112c78339--out-dirC:UsersLiuKangDesktopRUST
ust_to_c argetarmv7a-none-eabi
eleasedeps--targetarmv7a-none-eabi-Ldependency=C:UsersLiuKangDesktopRUS
5T
ust_to_c argetarmv7a-none-eabi
eleasedeps-Ldependency=C:UsersLiuKangDesktopRUST
ust_to_c arget
eleasedeps`
6Finishedrelease[optimized]target(s)in0.11s
运行
复制 rust 生成的库文件到 application 目录下。
1LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
2$scons-j6
3scons:ReadingSConscriptfiles...
4scons:donereadingSConscriptfiles.
5scons:warning:youdonotseemtohavethepywin32extensionsinstalled;
6parallel(-j)buildsmaynotworkreliablywithopenPythonfiles.
7File"D:softwareenv_released_1.2.0env oolsPython27Scriptsscons.py",line204,in<module>
8scons:Buildingtargets...
9scons:buildingassociatedVariantDirtargets:build
10LINKrtthread.elf
11arm-none-eabi-objcopy-Obinaryrtthread.elfrtthread.bin
12arm-none-eabi-sizertthread.elf
13textdatabssdechexfilename
14628812214890796721756b035crtthread.elf
15scons:donebuildingtargets.
16
17LiuKang@DESKTOP-538H6DED:
epogithub
t-threadspqemu-vexpress-a9
18$qemu.bat
19WARNING:Imageformatwasnotspecifiedfor'sd.bin'andprobingguessedraw.
20Automaticallydetectingtheformatisdangerousforrawimages,writeoperationsonblock0willberestricted.
21Specifythe'raw'formatexplicitlytoremovetherestrictions.
22
23|/
24-RT-ThreadOperatingSystem
25/|4.0.4buildJul282021
262006-2021Copyrightbyrt-threadteam
27lwIP-2.1.2initialized!
28[I/sal.skt]SocketAbstractionLayerinitializesuccess.
29[I/SDIO]SDcardcapacity65536KB.
30[I/SDIO]switchingcardtohighspeedfailed!
31thisisfromrust
32callrustsum(1,2)=3
33callrustsubtract(2,1)=1
34callrustmultiply(2,2)=4
35callrustdivide(4,2)=2
36msh/>
来源:
https://club.rt-thread.org/ask/article/2944.html
-
嵌入式
+关注
关注
5087文章
19148浏览量
306196 -
Rust
+关注
关注
1文章
229浏览量
6622
原文标题:手把手教你用 Rust 开发嵌入式
文章出处:【微信号:strongerHuang,微信公众号:strongerHuang】欢迎添加关注!文章转载请注明出处。
发布评论请先 登录
相关推荐
哪些专业适合学习嵌入式开发?
嵌入式开发必备-RK3562演示Linux常用系统查询命令(上)触觉智能出品
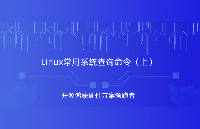
Made with KiCad(九十四):M5Pi Linux嵌入式开发板
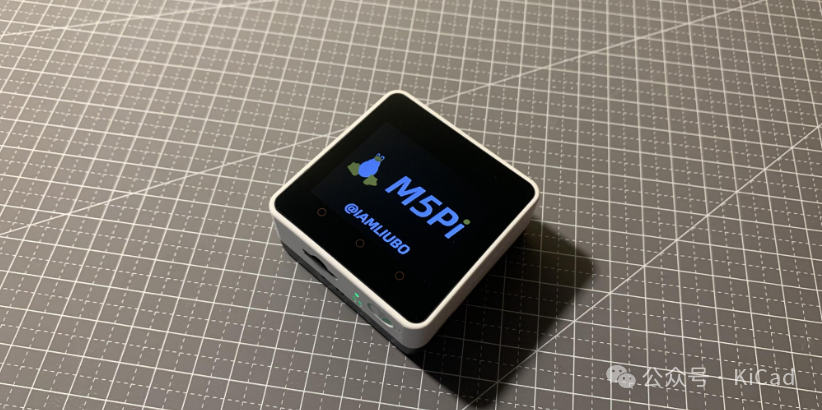
如何使用 RISC-V 进行嵌入式开发
基于Xilinx ZYNQ7000 FPGA嵌入式开发实战指南
零基础嵌入式开发学习路线
嵌入式开发常见问题排查
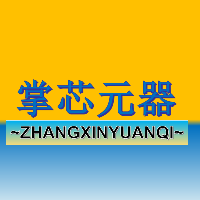
嵌入式开发常用软件有哪些?
聚焦嵌入式开发中的合规性工具、项目管理工具、版本迭代工具应用
嵌入式开发者的未来
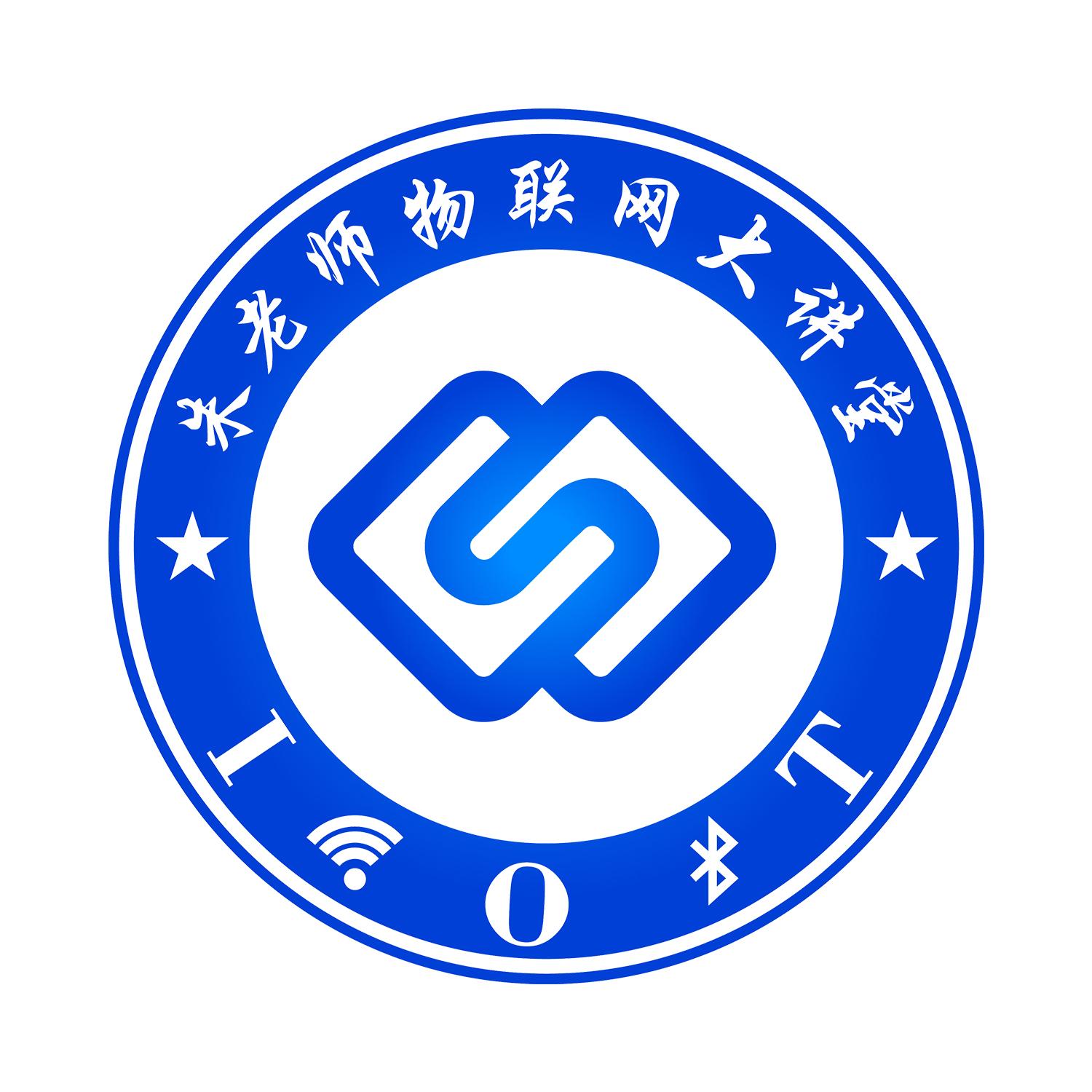
评论