# SwipeGesture
用于触发滑动事件,滑动速度大于100vp/s时可识别成功。
参数名称 | 参数类型 | 必填 | 参数描述 |
---|---|---|---|
fingers | number | 否 | 触发滑动的最少手指数,默认为1,最小为1指,最大为10指。 默认值:1 |
direction | SwipeDirection | 否 | 触发滑动手势的滑动方向。 默认值:SwipeDirection.All |
speed | number | 否 | 识别滑动的最小速度(默认为100VP/秒)。 默认值:100 |
SwipeDirection的三个枚举值
declare enum SwipeDirection {
/**
* Default.
* @since 8
*/
None,
/**
* Sliding horizontally.
* @since 8
*/
Horizontal,
/**
* Sliding Vertical
* @since 8
*/
Vertical,
/**
* Sliding in all directions.
* @since 8
*/
All
}
- All:所有方向。
- Horizontal:水平方向,手指滑动方向与x轴夹角小于45度时触发。
- Vertical:竖直方向,手指滑动方向与y轴夹角小于45度时触发。
- None:任何方向均不可触发。
事件
* Slide gesture recognition success callback.
* @since 8
*/
onAction(event: (event?: GestureEvent) => void): SwipeGestureInterface;
完整代码
@Entry
@Component
struct SwipeGestureExample {
@State rotateAngle: number = 0
@State speed: number = 1
build() {
Column() {
Column() {
Text("滑动 速度" + this.speed)
Text("滑动 角度" + this.rotateAngle)
}
.border({ width: 3 })
.width(300)
.height(200)
.margin(100)
.rotate({
angle: this.rotateAngle,})
// 单指竖直方向滑动时触发该事件
.gesture(
SwipeGesture({
fingers:2,
direction: SwipeDirection.All })
.onAction((event: GestureEvent) => {
this.speed = event.speed
this.rotateAngle = event.angle
})
)
}.width('100%')
}
}
RotationGesture
用于触发旋转手势事件,触发旋转手势的最少手指为2指,最大为5指,最小改变度数为1度。
数名称 | 参数类型 | 必填 | 参数描述 |
---|---|---|---|
fingers | number | 否 | 触发旋转的最少手指数, 最小为2指,最大为5指。 默认值:2 |
angle | number | 否 | 触发旋转手势的最小改变度数,单位为deg。 默认值:1 |
提供了四种事件
事件
- ** onActionStart(event: (event?: GestureEvent) => void):Rotation手势识别成功回调。**
- onActionUpdate(event: (event?: GestureEvent) => void):Rotation手势移动过程中回调。
- ** onActionEnd(event: (event?: GestureEvent) => void):Rotation手势识别成功,手指抬起后触发回调。**
- ** onActionCancel(event: () => void):Rotation手势识别成功,接收到触摸取消事件触发回调。**
* Pan gesture recognition success callback.
* @since 7
*/
onActionStart(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* Callback when the Pan gesture is moving.
* @since 7
*/
onActionUpdate(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized. When the finger is lifted, the callback is triggered.
* @since 7
*/
onActionEnd(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized and a callback is triggered when the touch cancel event is received.
* @since 7
*/
onActionCancel(event: () => void): RotationGestureInterface;
}
完整代码:
// xxx.ets
@Entry
@Component
struct RotationGestureExample {
@State angle: number = 0
@State rotateValue: number = 0
build() {
Column() {
Column() {
Text('旋转角度:' + this.angle)
}
.height(200)
.width(300)
.padding(20)
.border({ width: 3 })
.margin(80)
.rotate({ angle: this.angle })
// 双指旋转触发该手势事件
.gesture(
RotationGesture()
.onActionStart((event: GestureEvent) => {
console.info('Rotation start')
})
.onActionUpdate((event: GestureEvent) => {
this.angle = this.rotateValue + event.angle
})
.onActionEnd(() => {
this.rotateValue = this.angle
console.info('Rotation end')
}).onActionCancel(()=>{
console.info('Rotation onActionCancel')
})
)
}.width('100%')
}
}
用于触发滑动事件,滑动速度大于100vp/s时可识别成功。
参数名称 | 参数类型 | 必填 | 参数描述 |
---|---|---|---|
fingers | number | 否 | 触发滑动的最少手指数,默认为1,最小为1指,最大为10指。 默认值:1 |
direction | SwipeDirection | 否 | 触发滑动手势的滑动方向。 默认值:SwipeDirection.All |
speed | number | 否 | 识别滑动的最小速度(默认为100VP/秒)。 默认值:100 |
SwipeDirection的三个枚举值
declare enum SwipeDirection {
/**
* Default.
* @since 8
*/
None,
/**
* Sliding horizontally.
* @since 8
*/
Horizontal,
/**
* Sliding Vertical
* @since 8
*/
Vertical,
/**
* Sliding in all directions.
* @since 8
*/
All
}
- All:所有方向。
- Horizontal:水平方向,手指滑动方向与x轴夹角小于45度时触发。
- Vertical:竖直方向,手指滑动方向与y轴夹角小于45度时触发。
- None:任何方向均不可触发。
事件
* Slide gesture recognition success callback.
* @since 8
*/
onAction(event: (event?: GestureEvent) => void): SwipeGestureInterface;
完整代码
@Entry
@Component
struct SwipeGestureExample {
@State rotateAngle: number = 0
@State speed: number = 1
build() {
Column() {
Column() {
Text("滑动 速度" + this.speed)
Text("滑动 角度" + this.rotateAngle)
}
.border({ width: 3 })
.width(300)
.height(200)
.margin(100)
.rotate({
angle: this.rotateAngle,})
// 单指竖直方向滑动时触发该事件
.gesture(
SwipeGesture({
fingers:2,
direction: SwipeDirection.All })
.onAction((event: GestureEvent) => {
this.speed = event.speed
this.rotateAngle = event.angle
})
)
}.width('100%')
}
}
RotationGesture
用于触发旋转手势事件,触发旋转手势的最少手指为2指,最大为5指,最小改变度数为1度。
数名称 | 参数类型 | 必填 | 参数描述 |
---|---|---|---|
fingers | number | 否 | 触发旋转的最少手指数, 最小为2指,最大为5指。 默认值:2 |
angle | number | 否 | 触发旋转手势的最小改变度数,单位为deg。 默认值:1 |
提供了四种事件
事件
- ** onActionStart(event: (event?: GestureEvent) => void):Rotation手势识别成功回调。**
- onActionUpdate(event: (event?: GestureEvent) => void):Rotation手势移动过程中回调。
- ** onActionEnd(event: (event?: GestureEvent) => void):Rotation手势识别成功,手指抬起后触发回调。**
- ** onActionCancel(event: () => void):Rotation手势识别成功,接收到触摸取消事件触发回调。**
* Pan gesture recognition success callback.
* @since 7
*/
onActionStart(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* Callback when the Pan gesture is moving.
* @since 7
*/
onActionUpdate(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized. When the finger is lifted, the callback is triggered.
* @since 7
*/
onActionEnd(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized and a callback is triggered when the touch cancel event is received.
* @since 7
*/
onActionCancel(event: () => void): RotationGestureInterface;
}
完整代码:
// xxx.ets
@Entry
@Component
struct RotationGestureExample {
@State angle: number = 0
@State rotateValue: number = 0
build() {
Column() {
Column() {
Text('旋转角度:' + this.angle)
}
.height(200)
.width(300)
.padding(20)
.border({ width: 3 })
.margin(80)
.rotate({ angle: this.angle })
// 双指旋转触发该手势事件
.gesture(
RotationGesture()
.onActionStart((event: GestureEvent) => {
console.info('Rotation start')
})
.onActionUpdate((event: GestureEvent) => {
this.angle = this.rotateValue + event.angle
})
.onActionEnd(() => {
this.rotateValue = this.angle
console.info('Rotation end')
}).onActionCancel(()=>{
console.info('Rotation onActionCancel')
})
)
}.width('100%')
}
}
声明:本文内容及配图由入驻作者撰写或者入驻合作网站授权转载。文章观点仅代表作者本人,不代表电子发烧友网立场。文章及其配图仅供工程师学习之用,如有内容侵权或者其他违规问题,请联系本站处理。
举报投诉
-
手势识别
+关注
关注
8文章
225浏览量
47772 -
触摸
+关注
关注
7文章
198浏览量
64143 -
OpenHarmony
+关注
关注
25文章
3658浏览量
16145 -
OpenHarmony3.1
+关注
关注
0文章
11浏览量
614
发布评论请先 登录
相关推荐
红外手势识别方案 红外手势感应模块 红外识别红外手势识别
红外手势识别方案,适用于多种领域,如音响,可实现通过手势识别暂停,开始,上一首,下一首;智能家居,如电动窗帘,感应马桶等;电子产品,如台灯开关以及亮度的调节。
发表于 08-27 16:37
Android 手势识别
本帖最后由 kiter_rp 于 2014-9-11 14:23 编辑
总体来分析手势有关涉及到手势匹配相关的源码类之间的关系,如下图: 上图中的相关类简介:GestureLibrary:手势
发表于 09-11 14:22
使用SensorTile识别手势
你好, 我正在尝试使用SensorTile实现手势识别,开发我的固件我开始研究BlueMicrosystem2示例,因此我能够检测到简单的手势作为手腕的方向。现在我想要认识一些更复杂的手势,比如
发表于 09-10 17:18
手势识别控制器制作
目录智能家居硬件小制作(含源码)《手势识别控制器》基于PAJ7620手势模块、L298N驱动板、arduino介绍材料PAJ7620手势模块参数硬件连接库文件使用其他硬件制作手势识别控
发表于 09-07 06:45
HarmonyOS应用API手势方法-绑定手势方法
述:为组件绑定不同类型的手势事件,并设置事件的响应方法。Api:从API Version 7开始支持一、绑定手势识别:通过如下属性给组件绑定手势识别,手势识别成功后可以通过事件回调通知
发表于 11-23 15:53
HarmonyOS应用API手势方法-RotationGesture
描述:用于触发旋转手势事件,触发旋转手势的最少手指为2指,最大为5指,最小改变度数为1度。Api:从API Version 7开始支持接口:RotationGesture(value?: &
发表于 11-30 10:42
HarmonyOS应用API手势方法-RotationGesture
描述:用于触发旋转手势事件,触发旋转手势的最少手指为2指,最大为5指,最小改变度数为1度。Api:从API Version 7开始支持接口:RotationGesture(value?: &
发表于 11-30 10:43
HarmonyOS应用API手势方法-SwipeGesture
描述:用于触发滑动事件,滑动最小速度为100vp/s时识别成功。Api:从API Version 8开始支持接口:SwipeGesture(value?: { fingers
发表于 12-01 17:29
HarmonyOS/OpenHarmony(Stage模型)应用开发单一手势(三)
五、旋转手势(RotationGesture)
.RotationGesture(value?:{fingers?:number; angle?:number})
旋转手势用于触发旋转
发表于 09-06 14:14
HarmonyOS/OpenHarmony(Stage模型)应用开发组合手势(三)互斥识别
的触发条件为滑动距离达到5vp,先达到触发条件的手势触发。可以通过修改SwipeGesture和PanGesture的参数以达到不同的效果。
发表于 09-11 15:01
基于加锁机制的静态手势识别运动中的手势
基于 RGB-D( RGB-Depth)的静态手势识别的速度高于其动态手势识别,但是存在冗余手势和重复手势而导致识别准确性不高的问题。针对该问题,提出了一种基于加锁机制的静态
发表于 12-15 13:34
•0次下载
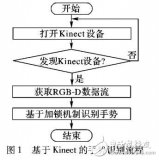
混合交互手势模型设计
分析了触控交互技术在移动手持设备及可穿戴设备的应用现状及存在的问题.基于交互动作的时间连续性及空间连续性。提出了将触控交互动作的接触面轨迹与空间轨迹相结合。同时具有空中手势及触控手势的特性及优点
发表于 12-26 11:15
•0次下载
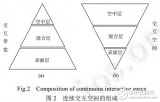
手势识别技术及其应用
手势识别技术是一种通过计算机视觉和人工智能技术来分析和识别人类手势动作的技术。它主要利用传感器、摄像头等设备捕捉手势信息,然后通过算法对捕捉到的手势信息进行处理和分析,从而实现对
车载手势识别技术的原理及其应用
车载手势识别技术是一种利用计算机视觉和人工智能技术来识别和理解驾驶员手势的技术。该技术通过使用传感器、摄像头等设备捕捉驾驶员的手势动作,然后通过算法对捕捉到的手势动作进行识别和分析,以
评论