在用到linux编程的时候,Makefile可以很好的帮助管理C语言工程,如何构建一个静态库,用一个很小的案例来说明。
首先准备需要的文件,以及文件中的内容,如下所示
$ cat test1.c
#include
int main()
{
printf("hello world\\n");
return 0;
}
这个.c文件非常简单,就是输出一个hello world。用gcc编译,就能输出。
`
: ~/Documents/clan/test1$ gcc test1.c
:~ /Documents/clan/test1$ tree
.
├── a.out
└── test1.c
0 directories, 3 files
:~/Documents/clan/test1$ ./a.out
hello world
现在换种方式实现,采用Makefile的形式,编辑Makefile的脚本
:~/Documents/clan/test1$ rm a.out
:~/Documents/clan/test1$ cat Makefile
test1 : test1.o
gcc -o test1 test1.o
test1.o : test1.c
gcc -c -o test1.o test1.c
clean :
rm -f test1 test1.o
编译Makefile文件,同样可以实现这个效果
:~/Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
:/Documents/clan/test1$ make/Documents/clan/test1$ tree
gcc -c -o test1.o test1.c
gcc -o test1 test1.o
:
.
├── Makefile
├── test1
├── test1.c
└── test1.o
0 directories, 4 files
:~/Documents/clan/test1$ ./test1
hello world
现在将产物删掉,方便后面的实验
: ~/Documents/clan/test1$ make clean
rm -f test1 test1.o
:~ /Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
现在回到gcc 编译的过程中,先编译得到.o文件,然后编译得到静态库文件,最后通过编译库文件,同样可以生成可执行文件
: ~/Documents/clan/test1$ gcc -c -o test1.o test1.c
:~ /Documents/clan/test1$ tree
.
├── Makefile
├── test1.c
└── test1.o
0 directories, 3 files
: ~/Documents/clan/test1$ ar -cr libtest1.a test1.o
:~ /Documents/clan/test1$ tree
.
├── libtest1.a
├── Makefile
├── test1.c
└── test1.o
0 directories, 4 files
: ~/Documents/clan/test1$ gcc libtest1.a
:~ /Documents/clan/test1$ tree
.
├── a.out
├── libtest1.a
├── Makefile
├── test1.c
└── test1.o
0 directories, 5 files
:~/Documents/clan/test1$ ./a.out
hello world
删除上述生成的文件
: ~/Documents/clan/test1$ rm a.out
:~ /Documents/clan/test1$ rm libtest1.a
: ~/Documents/clan/test1$ rm test1.o
:~ /Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
重新编辑Makefile文件
test1 : libtest1.a
gcc -o test1 libtest1.a
libtest1.a : test1.o
ar -cr libtest1.a test1.o
test1.o : test1.c
gcc -c -o test1.o test1.c
clean :
rm -f test1 test1.o libtest1.a
重新编译Makefile文件,然后执行,同样可以实现,这就是静态库的实现方式
: ~/Documents/clan/test1$ make
gcc -c -o test1.o test1.c
ar -cr libtest1.a test1.o
gcc -o test1 libtest1.a
:~ /Documents/clan/test1$ tree
.
├── libtest1.a
├── Makefile
├── test1
├── test1.c
└── test1.o
0 directories, 5 files
:~/Documents/clan/test1$ ./test1
hello world
上述方式,实现了一个非常简单的案例,这是在同一目录层级下实现的,如果涉及到多个目录层级呢?
├── func
│ ├── func.c
│ └── func.h
├── Makefile
└── test1.c
其中func.c文件的代码如下
#include "func.h"
int add(int a, int b){return a+b;}
func.h文件的代码
int add(int a, int b);
test.c文件的代码
#include
#include "func/func.h"
int main()
{
printf("hello world\\n");
printf("%d\\n",add(10,20));
return 0;
}
用gcc命令编译
然后修改Makefile文件
:~/Documents/clan/test1$ cat Makefile
test1 : test1.o func/func.o
gcc -o test1 test1.o func/func.o
test1.o : test1.c
gcc -c -o test1.o test1.c
func/func.o : func/func.c func/func.h
gcc -c -o func/func.o func/func.c
clean :
rm -f test1 test1.o func/func.o
编译所有文件,运行可执行文件,即可输出结果
:~/Documents/clan/test1$ tree
.
├── func
│ ├── func.c
│ └── func.h
├── Makefile
└── test1.c
1 directory, 4 files
: ~/Documents/clan/test1$ make
gcc -c -o test1.o test1.c
gcc -c -o func/func.o func/func.c
gcc -o test1 test1.o func/func.o
:~ /Documents/clan/test1$ tree
.
├── func
│ ├── func.c
│ ├── func.h
│ └── func.o
├── Makefile
├── test1
├── test1.c
└── test1.o
1 directory, 7 files
:~/Documents/clan/test1$ ./test1
hello world
30
要生成Makefile的静态库,只需要在这个基础上进行修改即可
test1 : libtest1.a func/libfunc.a
gcc -o test1 libtest1.a func/libfunc.a
libtest1.a : test1.o
ar -cr libtest1.a test1.o
func/libfunc.a : func/func.o
ar -cr func/libfunc.a func/func.o
test1.o : test1.c
gcc -c -o test1.o test1.c
func/func.o : func/func.c func/func.h
gcc -c -o func/func.o func/func.c
clean :
rm -f test1 test1.o libtest1.a func/libfunc.a func/func.o
这是一个非常简单的模板案例,静态库的编译过程比较简单,动态库相对复杂。
审核编辑:刘清
-
C语言
+关注
关注
180文章
7598浏览量
136210 -
gcc编译器
+关注
关注
0文章
78浏览量
3363 -
Linux编程
+关注
关注
0文章
5浏览量
614
发布评论请先 登录
相关推荐
学习C语言的目标和方法有哪些及C语言的关键字说明
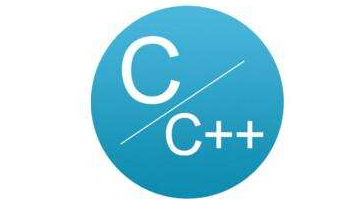
评论