今天的题目是 53. Maximum Subarray 最大子序和
Given an integer array nums, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Example:
Input: [-2,1,-3,4,-1,2,1,-5,4],
Output: 6
Explanation: [4,-1,2,1] has the largest sum = 6.
Follow up:
If you have figured out the O(n) solution, try coding another solution using the divide and conquer approach, which is more subtle.
给定一个整数数组 nums ,找到一个具有最大和的连续子数组(子数组最少包含一个元素),返回其最大和。
示例:
输入: [-2,1,-3,4,-1,2,1,-5,4],
输出: 6
解释: 连续子数组 [4,-1,2,1] 的和最大,为 6。
进阶:
如果你已经实现复杂度为 O(n) 的解法,尝试使用更为精妙的分治法求解。
Solutions:
class Solution:
def maxSubArray(self, nums: List[int]) -> int:
max_sum = nums[0]
lst = 0
# if(len(nums)==1): return nums[0]
'''
设置一个累加值,一个next_item值,一个max_sum值进行比较。
累加值是经过的数值累加的结果,next_item指示循环中的下一个新值,
max_sum用来保留全局最大,并做返回值。
'''
for next_item in nums:
lst = max(next_item,lst+next_item)
max_sum = max(max_sum,lst)
return max_sum
class Solution:
def maxSubArray(self, nums: List[int]) -> int:
'''
用DP的思想来解,并对数组进行原地修改,修改后的值等于该位置之前的最大累加和。
nums[0]不变,从nums[1]开始更新,对于i位置新值等于nums[i]和nums[i]+累加值
nums[i-1]中最大项。如果nums[i]小于0则累加后数值变小,经过max之后会被筛选掉。
最后返回nums数组中的最大值即可。
'''
for i in range(1, len(nums)):
nums[i] = max(nums[i], nums[i] + nums[i - 1])
return max(nums)
-
元素
+关注
关注
0文章
47浏览量
8442 -
连续
+关注
关注
0文章
16浏览量
8856 -
数组
+关注
关注
1文章
417浏览量
25956
发布评论请先 登录
相关推荐
请问数组定义全部是0,节点最大子节点数目是多少呢?
为什么架空输电线路的零序电抗大于其正序电抗?
What is the maximum temperatur
什么是maximum DSL speeds
零序保护的最大特点是什么_零序保护特点详解
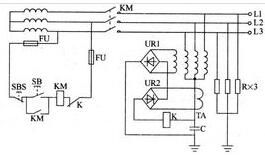
零序保护有方向性吗_零序保护的最大特点
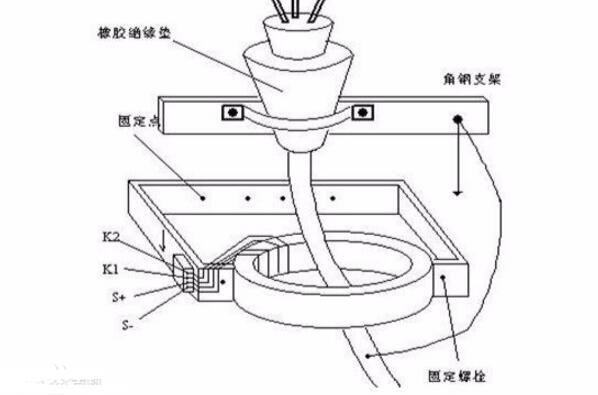
评论