4.硬件定时器的使用和学习
这里依然使用mdk的看法环境,使用mdk编译程序,下载程序
4.1配置使能硬件定时器2
4.2 编写定时器的测试函数
/*
程序清单:这是一个 hwtimer 设备使用例程
例程导出了 hwtimer_sample 命令到控制终端
命令调用格式:hwtimer_sample
程序功能:硬件定时器超时回调函数周期性的打印当前tick值,2次tick值之差换算为时间等同于定时时间值。
/
#include
#include
#define HWTIMER_DEV_NAME "time2" / 定时器名称 /
/ 定时器超时回调函数 /
static rt_err_t timeout_cb(rt_device_t dev, rt_size_t size)
{
rt_kprintf("this is hwtimer timeout callback fucntion!n");
rt_kprintf("tick is :%d !n", rt_tick_get());
return 0;
}
int hwtimer_sample(void)
{
rt_err_t ret = RT_EOK;
rt_hwtimerval_t timeout_s; / 定时器超时值 /
rt_device_t hw_dev = RT_NULL; / 定时器设备句柄 /
rt_hwtimer_mode_t mode; / 定时器模式 /
rt_uint32_t freq = 10000; / 计数频率 /
/ 查找定时器设备 /
hw_dev = rt_device_find(HWTIMER_DEV_NAME);
if (hw_dev == RT_NULL)
{
rt_kprintf("hwtimer sample run failed! can't find %s device!n", HWTIMER_DEV_NAME);
return RT_ERROR;
}
/ 以读写方式打开设备 /
ret = rt_device_open(hw_dev, RT_DEVICE_OFLAG_RDWR);
if (ret != RT_EOK)
{
rt_kprintf("open %s device failed!n", HWTIMER_DEV_NAME);
return ret;
}
/ 设置超时回调函数 /
rt_device_set_rx_indicate(hw_dev, timeout_cb);
/ 设置计数频率(若未设置该项,默认为1Mhz 或 支持的最小计数频率) /
rt_device_control(hw_dev, HWTIMER_CTRL_FREQ_SET, &freq);
/ 设置模式为周期性定时器(若未设置,默认是HWTIMER_MODE_ONESHOT)/
mode = HWTIMER_MODE_PERIOD;
ret = rt_device_control(hw_dev, HWTIMER_CTRL_MODE_SET, &mode);
if (ret != RT_EOK)
{
rt_kprintf("set mode failed! ret is :%dn", ret);
return ret;
}
/ 设置定时器超时值为5s并启动定时器 /
timeout_s.sec = 5; / 秒 /
timeout_s.usec = 0; / 微秒 /
if (rt_device_write(hw_dev, 0, &timeout_s, sizeof(timeout_s)) != sizeof(timeout_s))
{
rt_kprintf("set timeout value failedn");
return RT_ERROR;
}
/ 延时3500ms /
rt_thread_mdelay(3500);
/ 读取定时器当前值 /
rt_device_read(hw_dev, 0, &timeout_s, sizeof(timeout_s));
rt_kprintf("Read: Sec = %d, Usec = %dn", timeout_s.sec, timeout_s.usec);
return ret;
}
/ 导出到 msh 命令列表中 */
MSH_CMD_EXPORT(hwtimer_sample, hwtimer sample);
4.3测试函数,查看运行结果
4.4硬件定时器设备驱动框架学习
使用方法:
4.4.1实现定时器的各个操作函数
/*
- 定时器 初始化函数
- 定时器起始函数
- 定时器停止函数
- 定时器的计数值获取
- 定时器的控制函数
*/
struct rt_hwtimer_ops
{
void (*init)(struct rt_hwtimer_device *timer, rt_uint32_t state);
rt_err_t (*start)(struct rt_hwtimer_device *timer, rt_uint32_t cnt, rt_hwtimer_mode_t mode);
void (*stop)(struct rt_hwtimer_device *timer);
rt_uint32_t (*count_get)(struct rt_hwtimer_device *timer);
rt_err_t (*control)(struct rt_hwtimer_device *timer, rt_uint32_t cmd, void args);
};
4.4.2配置定时器的基本参数
/ 定时器特征描述 Timer Feature Information /
struct rt_hwtimer_info
{
rt_int32_t maxfreq; / 最大频率 the maximum count frequency timer support /
rt_int32_t minfreq; / 最小频率 the minimum count frequency timer support /
rt_uint32_t maxcnt; / 最大计数 值counter maximum value /
rt_uint8_t cntmode; / 计数方向 count mode (inc/dec) */
};
typedef struct rt_hwtimer_device
{
struct rt_device parent;//基本设备驱动框架
const struct rt_hwtimer_ops *ops;//定时器特有的操作函数
const struct rt_hwtimer_info info;//定时器相关的参数信息
rt_int32_t freq; / 用户设置的计数频率 counting frequency set by the user /
rt_int32_t overflow; / 定时器溢出 timer overflows /
float period_sec;
rt_int32_t cycles; / 溢出后将生成超时事件多少次 how many times will generate a timeout event after overflow /
rt_int32_t reload; / 重新加载循环(使用周期模式) reload cycles(using in period mode) /
rt_hwtimer_mode_t mode; / 计时模式(一次/周期) timing mode(oneshot/period) /
} rt_hwtimer_t;
4.4.3注册定时器的设备到驱动框架
/
定时器设备注册函数
*/
rt_err_t rt_device_hwtimer_register(rt_hwtimer_t *timer, const char *name, void *user_data);
4.4.4详细的定时器设备驱动相关
/*
Copyright (c) 2006-2023, RT-Thread Development Team
SPDX-License-Identifier: Apache-2.0
Change Logs:
Date Author Notes
/
#ifndef HWTIMER_H
#define HWTIMER_H
#include
#ifdef __cplusplus
extern "C" {
#endif
/ 定时器的控制命令类型 /
typedef enum
{
HWTIMER_CTRL_FREQ_SET = RT_DEVICE_CTRL_BASE(Timer) + 0x01, / 设置技术的频率set the count frequency /
HWTIMER_CTRL_STOP = RT_DEVICE_CTRL_BASE(Timer) + 0x02, / 停止定时器stop timer /
HWTIMER_CTRL_INFO_GET = RT_DEVICE_CTRL_BASE(Timer) + 0x03, / 获取计时器功能信息 get a timer feature information /
HWTIMER_CTRL_MODE_SET = RT_DEVICE_CTRL_BASE(Timer) + 0x04 / 设置定时器的工作模式 Setting the timing mode(oneshot/period) /
} rt_hwtimer_ctrl_t;
/ Timing Mode /
typedef enum
{
HWTIMER_MODE_ONESHOT = 0x01,//单次模式
HWTIMER_MODE_PERIOD//周期模式
} rt_hwtimer_mode_t;
/ Time Value /
typedef struct rt_hwtimerval
{
rt_int32_t sec; / 秒 second /
rt_int32_t usec; / 微秒 microsecond /
} rt_hwtimerval_t;
/ 计数的方向 /
#define HWTIMER_CNTMODE_UP 0x01 / increment count mode /
#define HWTIMER_CNTMODE_DW 0x02 / decreasing count mode /
struct rt_hwtimer_device;
/
- 定时器 初始化函数
- 定时器起始函数
- 定时器停止函数
- 定时器的计数值获取
- 定时器的控制函数
*/
struct rt_hwtimer_ops
{
void (*init)(struct rt_hwtimer_device *timer, rt_uint32_t state);
rt_err_t (*start)(struct rt_hwtimer_device *timer, rt_uint32_t cnt, rt_hwtimer_mode_t mode);
void (*stop)(struct rt_hwtimer_device *timer);
rt_uint32_t (*count_get)(struct rt_hwtimer_device *timer);
rt_err_t (*control)(struct rt_hwtimer_device *timer, rt_uint32_t cmd, void args);
};
/ 定时器特征描述 Timer Feature Information /
struct rt_hwtimer_info
{
rt_int32_t maxfreq; / 最大频率 the maximum count frequency timer support /
rt_int32_t minfreq; / 最小频率 the minimum count frequency timer support /
rt_uint32_t maxcnt; / 最大计数 值counter maximum value /
rt_uint8_t cntmode; / 计数方向 count mode (inc/dec) */
};
typedef struct rt_hwtimer_device
{
struct rt_device parent;//基本设备驱动框架
const struct rt_hwtimer_ops *ops;//定时器特有的操作函数
const struct rt_hwtimer_info info;//定时器相关的参数信息
rt_int32_t freq; / 用户设置的计数频率 counting frequency set by the user /
rt_int32_t overflow; / 定时器溢出 timer overflows /
float period_sec;
rt_int32_t cycles; / 溢出后将生成超时事件多少次 how many times will generate a timeout event after overflow /
rt_int32_t reload; / 重新加载循环(使用周期模式) reload cycles(using in period mode) /
rt_hwtimer_mode_t mode; / 计时模式(一次/周期) timing mode(oneshot/period) /
} rt_hwtimer_t;
/
定时器设备注册函数
*/
rt_err_t rt_device_hwtimer_register(rt_hwtimer_t *timer, const char *name, void *user_data);
/ 定时器回调函数 /
void rt_device_hwtimer_isr(rt_hwtimer_t *timer);
#ifdef __cplusplus
}
#endif
#endif
-
驱动器
+关注
关注
53文章
8252浏览量
146446 -
定时器
+关注
关注
23文章
3250浏览量
114892 -
计时器
+关注
关注
1文章
420浏览量
32727 -
回调函数
+关注
关注
0文章
87浏览量
11569 -
RT-Thread
+关注
关注
31文章
1291浏览量
40186
发布评论请先 登录
相关推荐
如何利用STM32L475开发板去处理定时器捕获模块应用程序
基于MCU的模块定时器的详细解析
基于cubemx的stm32开发之路(使用正点原子战舰V3开发板)——基本定时器的应用
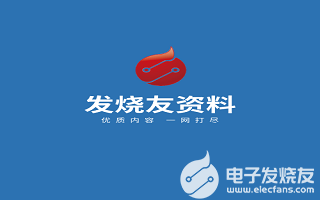
Linux驱动开发高精度定时器的精度测量评测
【合宙Air105开发板试用体验】小小定时器,能有大作用!
英飞凌开发板模块评测任务大挑战-SPI驱动测试
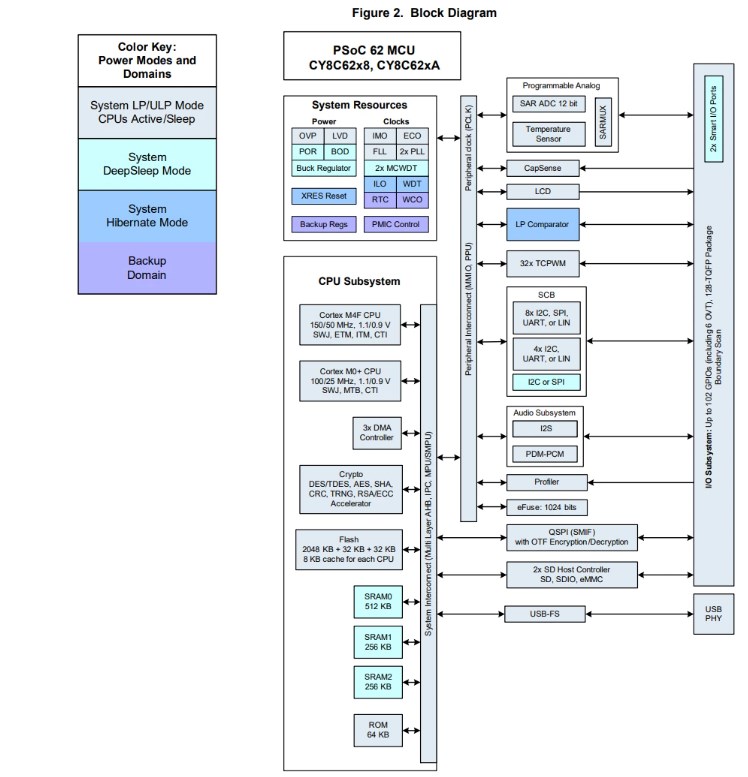
评论