【鸿蒙】鸿蒙如何进行数据解析
【问题描述】有时候我们从服务器获取是 xml 格式数据,我们需要将 xml 转化成 model 对象,该如何使用呢?下面举个例子说明一下,将分以下几步进行
1.准备条件 创建xml文件,创建model对象,构建界面
2.数据进行解析操作(重点)
3.运行效果
第一步准备条件 创建 xml 文件,创建 model 对象,构建界面
1.1 在 rawfile 新建 xml 文件,代码如下
< ?xml version="1.0" encoding="utf-8"? >
< note >
< to >George< /to >
< from >John< /from >
< heading >Reminder< /heading >
Don't forget the meeting!< /body > < /note >
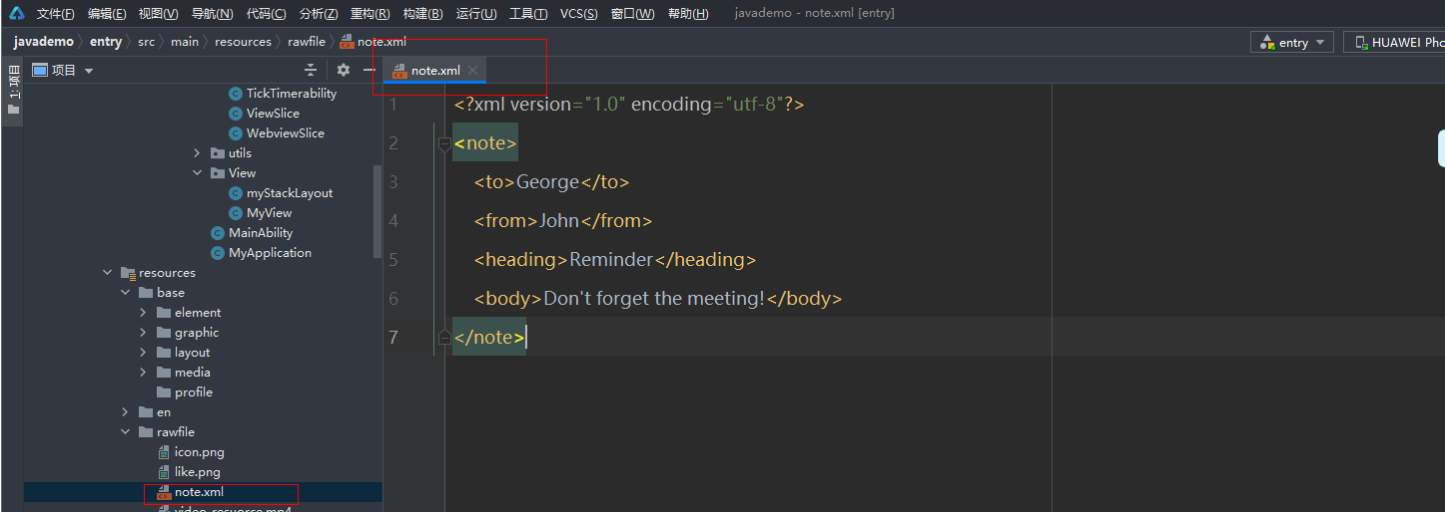
1.2 新建 class model 代码如下
package com.harmony.alliance.mydemo.model;
public class note {
private String from;
private String heading;
private String body;
private String to;
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public String getBody() {
return body;
}
public void setBody(String body) {
this.body = body;
}
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public String getHeading() {
return heading;
}
public void setHeading(String heading) {
this.heading = heading;
}
@Override
public String toString() {
return "note{" +
"from='" + from + ''' +
", heading='" + heading + ''' +
", body='" + body + ''' +
", to='" + to + ''' +
'}';
}
}
1.3 新建数据的 abilityslice,xml 如下
< ?xml version="1.0" encoding="utf-8"? >
< DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_parent"
ohos:width="match_parent"
ohos:orientation="vertical" >
< Text
ohos:id="$+id:mStartParse"
ohos:height="100vp"
ohos:width="match_parent"
ohos:text="开始解析"
ohos:text_size="20fp"
ohos:text_color="#ffffff"
ohos:text_alignment="center"
ohos:background_element="#ed6262"/ >
< Text
ohos:id="$+id:mTo"
ohos:height="100fp"
ohos:width="match_parent"
ohos:text_size="20fp"
ohos:text_color="#ed6262"
ohos:background_element="#ffffff"/ >
< Text
ohos:id="$+id:mfrom"
ohos:height="100fp"
ohos:width="match_parent"
ohos:text_size="20fp"
ohos:text_color="#ed6262"
ohos:background_element="#ffffff"/ >
< Text
ohos:id="$+id:mheading"
ohos:height="100fp"
ohos:width="match_parent"
ohos:text_size="20fp"
ohos:text_color="#ed6262"
ohos:background_element="#ffffff"/ >
< Text
ohos:id="$+id:mbody"
ohos:height="100fp"
ohos:width="match_parent"
ohos:text_size="20fp"
ohos:text_color="#ed6262"
ohos:background_element="#ffffff"/ >
< /DirectionalLayout >
界面效果如下
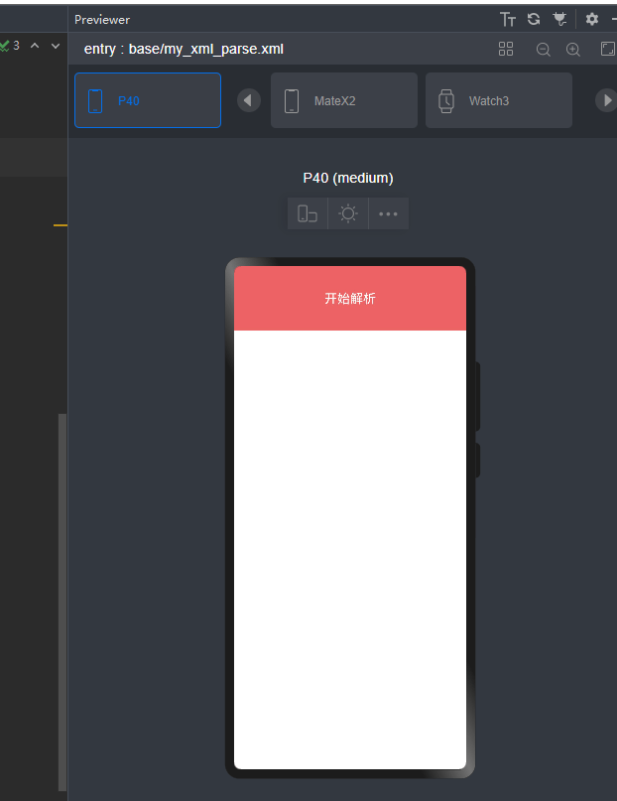
第二步数据解析 2.1 参考资料如下
SAXParser
https://developer.harmonyos.com/cn/docs/documentation/doc-references/saxparser-0000001060039145
XMLReader https://developer.harmonyos.com/cn/docs/documentation/doc-references/xmlreader-0000001060837300
在上述四个接口中,最重要的就是 ContentHandler 这个接口,下面是对这个接口方法的说明:
// 设置一个可以定位文档内容事件发生位置的定位器对象
public void setDocumentLocator(Locator locator)
// 用于处理文档解析开始事件
public void startDocument()throws SAXException
// 处理元素开始事件,从参数中可以获得元素所在名称空间的 uri,元素名称,属性类表等信息
public void startElement(String namespacesURI , String localName , String qName , Attributes atts) throws SAXException
// 处理元素结束事件,从参数中可以获得元素所在名称空间的 uri,元素名称等信息
public void endElement(String namespacesURI , String localName , String qName) throws SAXException
// 处理元素的字符内容,从参数中可以获得内容
public void characters(char[] ch , int start , int length) throws SAXException
新建 SaxHelper 代码如下
package com.harmony.alliance.mydemo.model;
import ohos.org.xml.sax.Attributes;
import ohos.org.xml.sax.SAXException;
import ohos.org.xml.sax.helpers.DefaultHandler;
/**
* Created by Jay on 2015/9/8 0008.
*/
public class SaxHelper extends DefaultHandler {
//当前解析的元素标签
private String tagName = null;
private note mNote;
/**
* 当读取到文档开始标志是触发,通常在这里完成一些初始化操作
*/
@Override
public void startDocument() throws SAXException {
mNote=new note();
}
/**
* 读到一个开始标签时调用,第二个参数为标签名,最后一个参数为属性数组
*/
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException {
System.err.println("####===>>"+localName);
this.tagName = localName;
}
/**
* 读到到内容,第一个参数为字符串内容,后面依次为起始位置与长度
*/
@Override
public void characters(char[] ch, int start, int length) throws SAXException {
//判断当前标签是否有效
if (this.tagName != null) {
String data = new String(ch, start, length);
//读取标签中的内容
if (this.tagName.equals("to")) {
mNote.setTo(data);
} else if (this.tagName.equals("from")) {
mNote.setFrom(data);
}else if(this.tagName.equals("heading")){
mNote.setHeading(data);
}else if(this.tagName.equals("body")){
mNote.setBody(data);
}
}
}
/**
* 处理元素结束时触发,这里将对象添加到结合中
*/
@Override
public void endElement(String uri, String localName, String qName) throws SAXException {
this.tagName = null;
}
/**
* 读取到文档结尾时触发,
*/
@Override
public void endDocument() throws SAXException {
super.endDocument();
System.err.println("#####===>>"+mNote.toString());
// Log.i("SAX", "读取到文档尾,xml解析结束");
}
public note getmNote() {
return mNote;
}
}
AbilitySlice 代码如下
package com.harmony.alliance.mydemo.slice;
import com.harmony.alliance.mydemo.ResourceTable;
import com.harmony.alliance.mydemo.model.SaxHelper;
import com.harmony.alliance.mydemo.model.note;
import ohos.aafwk.ability.AbilitySlice;
import ohos.aafwk.content.Intent;
import ohos.agp.components.Component;
import ohos.agp.components.Text;
import ohos.javax.xml.parsers.SAXParser;
import ohos.javax.xml.parsers.SAXParserFactory;
import ohos.org.xml.sax.InputSource;
import ohos.org.xml.sax.XMLReader;
import java.io.InputStream;
import java.util.Locale;
public class myXmlParseAbilitySlice extends AbilitySlice {
private Text mStartParse,mTo,mfrom,mheading,mbody;
@Override
protected void onStart(Intent intent) {
super.onStart(intent);
setUIContent(ResourceTable.Layout_my_xml_parse);
mStartParse= (Text) findComponentById(ResourceTable.Id_mStartParse);
mTo= (Text) findComponentById(ResourceTable.Id_mTo);
mfrom= (Text) findComponentById(ResourceTable.Id_mfrom);
mheading= (Text) findComponentById(ResourceTable.Id_mheading);
mbody= (Text) findComponentById(ResourceTable.Id_mbody);
mStartParse.setClickedListener(new Component.ClickedListener() {
@Override
public void onClick(Component component) {
try {
String rawFilePath = "note.xml";
String filePath = String.format(Locale.ROOT, "assets/entry/resources/rawfile/%s", rawFilePath);
InputStream is = this.getClass().getClassLoader().getResourceAsStream(filePath);
InputSource is2=new InputSource(is);
SaxHelper ss = new SaxHelper();
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setNamespaceAware(true);
SAXParser parser = factory.newSAXParser();
XMLReader xmlReader=parser.getXMLReader();
xmlReader.setContentHandler(ss);
xmlReader.parse(is2);
note mNote= ss.getmNote();
mTo.setText("to: "+mNote.getTo());
mfrom.setText("From: "+mNote.getFrom());
mheading.setText("heading: "+mNote.getHeading());
mbody.setText("body: "+mNote.getBody());
is.close();
}catch (Exception e){
e.printStackTrace();
}
}
});
}
}
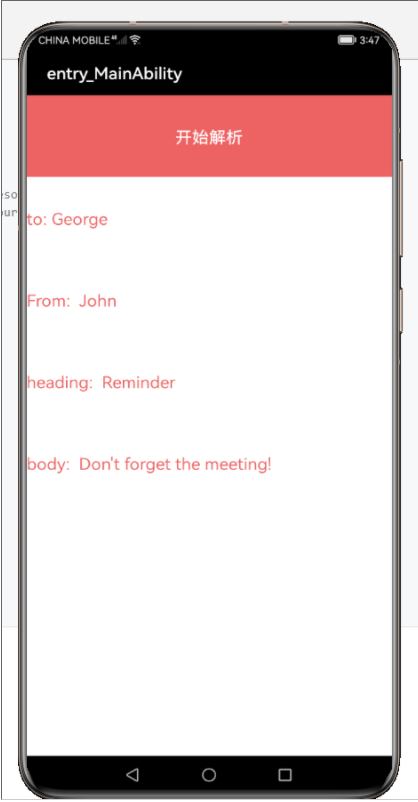
审核编辑 黄宇
-
XML
+关注
关注
0文章
186浏览量
33021 -
Model
+关注
关注
0文章
338浏览量
25004 -
鸿蒙
+关注
关注
57文章
2301浏览量
42668 -
HarmonyOS
+关注
关注
79文章
1965浏览量
29953
发布评论请先 登录
相关推荐
XML在HarmonyOS中的生成,解析与转换(下)
【HarmonyOS IPC 试用连载 】鸿蒙系统初步了解
【HarmonyOS HiSpark AI Camera试用连载 】鸿蒙JS UI介绍
请教鸿蒙应用开发JAVA UI 框架ProgressBar或者RoundProgressBar怎么实现滑动调节
基于HarmonyOS Java UI使用元数据绑定框架实现UI和数据源的绑定
基于HarmonyOS Java UI,实现常见组件或者布局
鸿蒙应用开发入门资料合集
Java解析XML的一种数据绑定技术
java教程之GUI如何进行事件处理
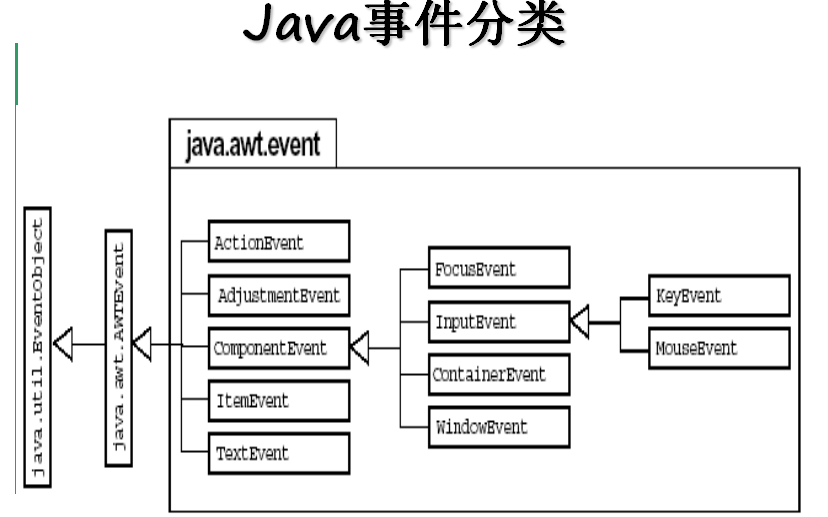
鸿蒙版微信聊天UI效果实现!
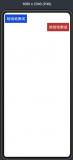
评论