匿名密钥证明(C/C++)
在使用本功能时,需确保网络通畅。
在CMake脚本中链接相关动态库
target_link_libraries(entry PUBLIC libhuks_ndk.z.so)
开发步骤
- 确定密钥别名keyAlias,密钥别名最大长度为64字节;
- 初始化参数集:通过[OH_Huks_InitParamSet]、[OH_Huks_AddParams]、[OH_Huks_BuildParamSet]构造参数集paramSet,参数集中必须包含[OH_Huks_KeyAlg],[OH_Huks_KeySize],[OH_Huks_KeyPurpose]属性;
- 将密钥别名与参数集作为参数传入[OH_Huks_AnonAttestKeyItem]方法中,即可证明密钥。
#include "huks/native_huks_api.h"
#include "huks/native_huks_param.h"
#include < string.h >
OH_Huks_Result InitParamSet(
struct OH_Huks_ParamSet **paramSet,
const struct OH_Huks_Param *params,
uint32_t paramCount)
{
OH_Huks_Result ret = OH_Huks_InitParamSet(paramSet);
if (ret.errorCode != OH_HUKS_SUCCESS) {
return ret;
}
ret = OH_Huks_AddParams(*paramSet, params, paramCount);
if (ret.errorCode != OH_HUKS_SUCCESS) {
OH_Huks_FreeParamSet(paramSet);
return ret;
}
ret = OH_Huks_BuildParamSet(paramSet);
if (ret.errorCode != OH_HUKS_SUCCESS) {
OH_Huks_FreeParamSet(paramSet);
return ret;
}
return ret;
}
static uint32_t g_size = 4096;
static uint32_t CERT_COUNT = 4;
void FreeCertChain(struct OH_Huks_CertChain *certChain, const uint32_t pos)
{
if (certChain == nullptr || certChain- >certs == nullptr) {
return;
}
for (uint32_t j = 0; j < pos; j++) {
if (certChain- >certs[j].data != nullptr) {
free(certChain- >certs[j].data);
certChain- >certs[j].data = nullptr;
}
}
if (certChain- >certs != nullptr) {
free(certChain- >certs);
certChain- >certs = nullptr;
}
}
int32_t ConstructDataToCertChain(struct OH_Huks_CertChain *certChain)
{
if (certChain == nullptr) {
return OH_HUKS_ERR_CODE_ILLEGAL_ARGUMENT;
}
certChain- >certsCount = CERT_COUNT;
certChain- >certs = (struct OH_Huks_Blob *)malloc(sizeof(struct OH_Huks_Blob) * (certChain- >certsCount));
if (certChain- >certs == nullptr) {
return OH_HUKS_ERR_CODE_INTERNAL_ERROR;
}
for (uint32_t i = 0; i < certChain- >certsCount; i++) {
certChain- >certs[i].size = g_size;
certChain- >certs[i].data = (uint8_t *)malloc(certChain- >certs[i].size);
if (certChain- >certs[i].data == nullptr) {
FreeCertChain(certChain, i);
return OH_HUKS_ERR_CODE_ILLEGAL_ARGUMENT;
}
}
return 0;
}
static struct OH_Huks_Param g_genAnonAttestParams[] = {
{ .tag = OH_HUKS_TAG_ALGORITHM, .uint32Param = OH_HUKS_ALG_RSA },
{ .tag = OH_HUKS_TAG_KEY_SIZE, .uint32Param = OH_HUKS_RSA_KEY_SIZE_2048 },
{ .tag = OH_HUKS_TAG_PURPOSE, .uint32Param = OH_HUKS_KEY_PURPOSE_VERIFY },
{ .tag = OH_HUKS_TAG_DIGEST, .uint32Param = OH_HUKS_DIGEST_SHA256 },
{ .tag = OH_HUKS_TAG_PADDING, .uint32Param = OH_HUKS_PADDING_PSS },
{ .tag = OH_HUKS_TAG_BLOCK_MODE, .uint32Param = OH_HUKS_MODE_ECB },
};
#define CHALLENGE_DATA "hi_challenge_data"
static struct OH_Huks_Blob g_challenge = { sizeof(CHALLENGE_DATA), (uint8_t *)CHALLENGE_DATA };
static napi_value AnonAttestKey(napi_env env, napi_callback_info info)
{
/* 1.确定密钥别名 */
struct OH_Huks_Blob genAlias = {
(uint32_t)strlen("test_anon_attest"),
(uint8_t *)"test_anon_attest"
};
static struct OH_Huks_Param g_anonAttestParams[] = {
{ .tag = OH_HUKS_TAG_ATTESTATION_CHALLENGE, .blob = g_challenge },
{ .tag = OH_HUKS_TAG_ATTESTATION_ID_ALIAS, .blob = genAlias },
};
struct OH_Huks_ParamSet *genParamSet = nullptr;
struct OH_Huks_ParamSet *anonAttestParamSet = nullptr;
OH_Huks_Result ohResult;
OH_Huks_Blob certs = { 0 };
OH_Huks_CertChain certChain = { &certs, 0 };
do {
/* 2.初始化密钥参数集 */
ohResult = InitParamSet(&genParamSet, g_genAnonAttestParams, sizeof(g_genAnonAttestParams) / sizeof(OH_Huks_Param));
if (ohResult.errorCode != OH_HUKS_SUCCESS) {
break;
}
ohResult = InitParamSet(&anonAttestParamSet, g_anonAttestParams, sizeof(g_anonAttestParams) / sizeof(OH_Huks_Param));
if (ohResult.errorCode != OH_HUKS_SUCCESS) {
break;
}
ohResult = OH_Huks_GenerateKeyItem(&genAlias, genParamSet, nullptr);
if (ohResult.errorCode != OH_HUKS_SUCCESS) {
break;
}
(void)ConstructDataToCertChain(&certChain);
/* 3.证明密钥 */
ohResult = OH_Huks_AttestKeyItem(&genAlias, attestParamSet, &certChain);
} while (0);
FreeCertChain(&certChain, CERT_COUNT);
OH_Huks_FreeParamSet(&genParamSet);
OH_Huks_FreeParamSet(&anonAttestParamSet);
(void)OH_Huks_DeleteKeyItem(&genAlias, NULL);
napi_value ret;
napi_create_int32(env, ohResult.errorCode, &ret);
return ret;
}
审核编辑 黄宇
声明:本文内容及配图由入驻作者撰写或者入驻合作网站授权转载。文章观点仅代表作者本人,不代表电子发烧友网立场。文章及其配图仅供工程师学习之用,如有内容侵权或者其他违规问题,请联系本站处理。
举报投诉
-
C++
+关注
关注
21文章
2094浏览量
73442 -
鸿蒙
+关注
关注
57文章
2301浏览量
42665
发布评论请先 登录
相关推荐
鸿蒙开发:Universal Keystore Kit密钥管理服务 密钥导入介绍及算法规格
如果业务在HUKS外部生成密钥(比如应用间协商生成、服务器端生成),业务可以将密钥导入到HUKS中由HUKS进行管理。密钥一旦导入到HUKS
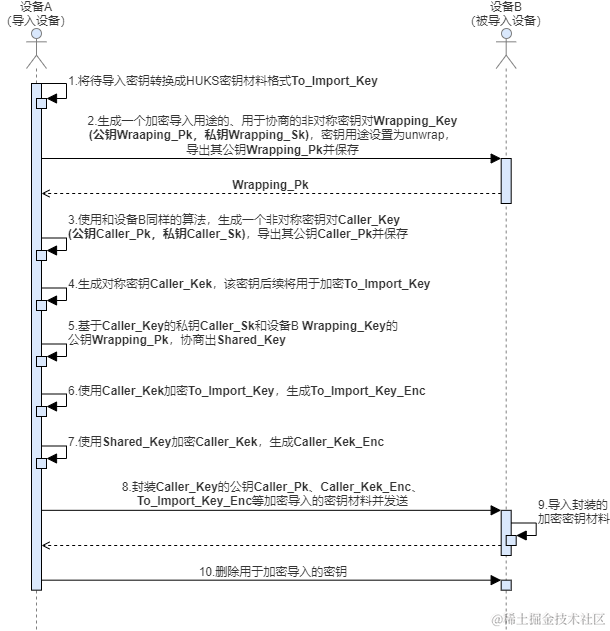
鸿蒙开发:Universal Keystore Kit 密钥管理服务 密钥协商 C、C++
以协商密钥类型为ECDH,并密钥仅在HUKS内使用为例,完成密钥协商。具体的场景介绍及支持的算法规格,请参考[密钥生成支持的算法]。
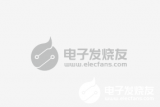
鸿蒙开发:Universal Keystore Kit 密钥管理服务 HMAC ArkTS
HMAC是密钥相关的哈希运算消息认证码(Hash-based Message Authentication Code),是一种基于Hash函数和密钥进行消息认证的方法。
鸿蒙开发:Universal Keystore Kit 密钥管理服务 HMAC C、C++
HMAC是密钥相关的哈希运算消息认证码(Hash-based Message Authentication Code),是一种基于Hash函数和密钥进行消息认证的方法。
鸿蒙开发:Universal Keystore Kit密钥管理服务 密钥证明介绍及算法规格
HUKS为密钥提供合法性证明能力,主要应用于非对称密钥的公钥的证明。
鸿蒙开发:Universal Keystore Kit 密钥管理服务 获取密钥属性C C++
HUKS提供了接口供业务获取指定密钥的相关属性。在获取指定密钥属性前,需要确保已在HUKS中生成或导入持久化存储的密钥。
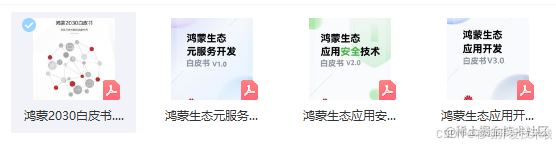
鸿蒙开发:Universal Keystore Kit 密钥管理服务 密钥导出 C C++
业务需要获取持久化存储的非对称密钥的公钥时使用,当前支持ECC/RSA/ED25519/X25519的公钥导出。
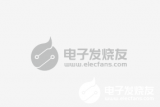
评论