STK600 之 Atmega128硬件I2C 读写高精度时钟芯片DS3231函数
STK600 用于程序的下载 连接JTAG口至mega128目标板即可
//-----------------------------------------------------------------------------
unsigned char DS3231_DATA[19] = {0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,};
unsigned char Date_Data[14] = {0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00};
unsigned char Data_temp[6] = {0x00,0x00,0x00,0x00,0x00,0x00};
unsigned char Buffer_Data[20] = {0x21,0x22,0x23,0x24,0x25,0x26,0x27,0x28,0x29,0x2A,0x2B,
0x2C,0x2D,0x2E,0x2F,0x30,0x31,0x32,0x33,0x34,};
void DS3221_initial(void)
{
DS3231_RESETH;
DS3231_RESETL;
delay_ms(300);
DS3231_RESETH;
}
void twi_start_state(void)
{
TWCR = TWCR | 0xA4;
twi_intcheck();
}
void twi_stop_state(void)
{
TWCR = TWCR | 0x94;
TWCR = TWCR | 0x84;
}
void twi_slaw(unsigned char address)
{
address = address << 1;
TWDR = address;
TWCR = TWCR & 0xDF;
TWCR = TWCR | 0x84;
}
void twi_slar(unsigned char address)
{
address = address << 1;
address = address | 0x01;
TWDR = address;
TWCR = TWCR & 0xDF;
TWCR = TWCR | 0x84;
}
void twi_wordadd_write(unsigned char address)
{
TWDR = address;
TWCR = TWCR & 0xDF;
TWCR = TWCR | 0x84;
}
void twi_datawrite(unsigned char data)
{
TWDR = data;
TWCR = TWCR | 0x84;
}
unsigned char twi_dataread(void)
{
unsigned char temp_a;
twi_intcheck();
temp_a = TWDR;
return temp_a;
}
void twi_MT(unsigned char sladdress,unsigned char wordaddress,unsigned char *ds3231data,unsigned char datalength)
{
unsigned char temp_a;
unsigned char temp_b;
twi_start_state();
twi_intcheck();
twi_slaw(sladdress);
twi_intcheck();
twi_wordadd_write(wordaddress);
twi_intcheck();
for(temp_a = 0;temp_a < datalength;temp_a++)
{
temp_b = *ds3231data;
twi_datawrite(temp_b);
++ds3231data;
twi_intcheck();
}
twi_intclear();
twi_stop_state();
}
void twi_MR(unsigned char sladdress,
unsigned char wordaddress,
unsigned char *ds3231data,
unsigned char datalength)
{
unsigned char temp_a;
twi_start_state();
twi_intcheck();
twi_slar(sladdress);
twi_intcheck();
twi_intclear();
for(temp_a = 0;temp_a < datalength;temp_a++)
{
*ds3231data = twi_dataread();
++ds3231data;
twi_intclear();
}
twi_stop_state();
}
void twi_MTR(unsigned char sladdress,
unsigned char wordaddress,
unsigned char *ds3231data,
unsigned char datalength)
{
unsigned char temp_a;
twi_start_state();
twi_intcheck();
twi_slaw(sladdress);
twi_intcheck();
twi_wordadd_write(wordaddress);
twi_intcheck();
twi_start_state();
twi_intcheck();
twi_slar(sladdress);
twi_intcheck();
twi_intclear();
for(temp_a = 0;temp_a < datalength;temp_a++)
{
*ds3231data = twi_dataread();
++ds3231data;
if(temp_a < (datalength - 1))
{
twi_intclear();
}
}
twi_intcheck();
TWCR = TWCR & 0xBF;
twi_stop_state();
TWCR = 0x44;
}
void twi_intcheck(void)
{
unsigned char temp_a;
temp_a = TWCR & 0x80;
while(temp_a == 0x00)
{
temp_a = TWCR & 0x80;
}
}
void twi_intclear(void)
{
TWCR = TWCR | 0x84;
}
void DS3231toDate(unsigned char *ds3231data,unsigned char *Datedata)
{
unsigned char temp_a;
for(temp_a = 0;temp_a < 7;temp_a++)
{
*Datedata = *ds3231data & 0x0F;
++Datedata;
*Datedata = *ds3231data >> 4;
++Datedata;
++ds3231data;
}
temp_a = 0;
}
void DS3231TD_set(unsigned char year,
unsigned char month,
unsigned char date,
unsigned char day,
unsigned char hour,
unsigned char minute,
unsigned char second,
unsigned char time_import,
unsigned char *ds3231data)
{
*ds3231data = second;
++ds3231data;
*ds3231data = minute;
++ds3231data;
if(time_import == Time12)
{
if(hour > 0x12)
{
hour = hour - 0x12;
}
else;
*ds3231data = hour | 0x40;
}
else
{
*ds3231data = hour & 0xBF;
}
++ds3231data;
*ds3231data = day;
++ds3231data;
*ds3231data = date;
++ds3231data;
*ds3231data = month;
++ds3231data;
*ds3231data = year;
twi_MT(DS3231address,0x00,&DS3231_DATA[0],7);
}
void temp_convert(unsigned char *temp_data,
unsigned char *ds3231data)
{
unsigned char temp_b;
temp_b = *ds3231data;
if((temp_b & 0x80) > 0)
{*temp_data = negative;}
else
{*temp_data = positive;}
temp_b = temp_b << 1;
temp_b = temp_b >> 1;
++temp_data;
*temp_data = temp_b / 100;
++temp_data;
*temp_data = (temp_b % 100) / 10;
++temp_data;
*temp_data = (temp_b % 100) % 10;
++ds3231data;
temp_b = *ds3231data;
temp_b = temp_b >> 6;
temp_b = temp_b * 25;
++temp_data;
*temp_data = temp_b / 10;
++temp_data;
*temp_data = temp_b % 10;
}
-
DS3231
+关注
关注
2文章
51浏览量
23840 -
时钟芯片
+关注
关注
2文章
249浏览量
39884
发布评论请先 登录
相关推荐
RISC V的I2C操作
TLV320AIC3263 i2c无法进行通信,通过i2c的读写函数,读写寄存器失败怎么解决?
具有兼容 SMBus 和 I2C 接口的 TMP116 高精度、低功耗数字温度传感器数据表
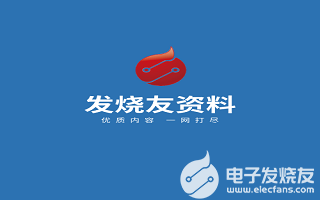
TMP117 具有 SMBus™ 和I2C兼容接口的高精度、低功耗数字温度传感器数据表
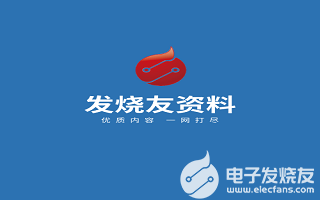
TMAG5173-Q1具有I2C接口的高精度3D霍尔效应传感器数据表
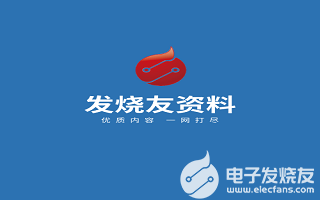
DS3231 RTC模块的I2C通信失败的原因?怎么解决?
ESP32C3 I2C no ack无应答怎么解决?
STM32F103利用软件模拟I2C读写EEPROM,超过385个字节就读写不了的原因?
什么是I2C协议 I2C总线的控制逻辑
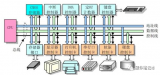
I2C接口称重采集单元

评论