资料介绍
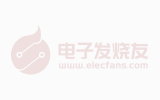
Table of Contents
SSM2602 Sound CODEC Linux Driver
Supported Devices
Evaluation Boards
Reference Circuits
Source Code
Status
Files
Function | File |
---|---|
driver | sound/soc/codecs/ssm2602.c |
include | sound/soc/codecs/ssm2602.h |
Example device initialization
For compile time configuration, it’s common Linux practice to keep board- and application-specific configuration out of the main driver file, instead putting it into the board support file.
For devices on custom boards, as typical of embedded and SoC-(system-on-chip) based hardware, Linux uses platform_data to point to board-specific structures describing devices and how they are connected to the SoC. This can include available ports, chip variants, preferred modes, default initialization, additional pin roles, and so on. This shrinks the board-support packages (BSPs) and minimizes board and application specific #ifdefs in drivers.
SPI
SPI can be used for the SSM2602 if in SPI mode (MODE pin set to 1).
Declaring SPI slave devices
Unlike PCI or USB devices, SPI devices are not enumerated at the hardware level. Instead, the software must know which devices are connected on each SPI bus segment, and what slave selects these devices are using. For this reason, the kernel code must instantiate SPI devices explicitly. The most common method is to declare the SPI devices by bus number.
This method is appropriate when the SPI bus is a system bus, as in many embedded systems, wherein each SPI bus has a number which is known in advance. It is thus possible to pre-declare the SPI devices that inhabit this bus. This is done with an array of struct spi_board_info, which is registered by calling spi_register_board_info().
For more information see: Documentation/spi/spi-summary
static struct spi_board_info board_spi_board_info[] __initdata = { [--snip--] { .modalias = "ssm2602", .max_speed_hz = 25000000, /* max spi clock (SCK) speed in HZ */ .bus_num = 0, .chip_select = GPIO_PF10 + MAX_CTRL_CS, /* CS, change it for your board */ .mode = SPI_MODE_3, }, [--snip--] };
static int __init board_init(void) { [--snip--] spi_register_board_info(board_spi_board_info, ARRAY_SIZE(board_spi_board_info)); [--snip--] return 0; } arch_initcall(board_init);
I2C
I2C can be used for the SSM2602, SSM2603, SSM2604. For the SSM2602 make sure that it is in I2C mode (MODE pin set to 0).
Declaring I2C devices
Unlike PCI or USB devices, I2C devices are not enumerated at the hardware level. Instead, the software must know which devices are connected on each I2C bus segment, and what address these devices are using. For this reason, the kernel code must instantiate I2C devices explicitly. There are different ways to achieve this, depending on the context and requirements. However the most common method is to declare the I2C devices by bus number.
This method is appropriate when the I2C bus is a system bus, as in many embedded systems, wherein each I2C bus has a number which is known in advance. It is thus possible to pre-declare the I2C devices that inhabit this bus. This is done with an array of struct i2c_board_info, which is registered by calling i2c_register_board_info().
So, to enable such a driver one need only edit the board support file by adding an appropriate entry to i2c_board_info.
For more information see: Documentation/i2c/instantiating-devices
static struct i2c_board_info __initdata bfin_i2c_board_info[] = { [--snip--] { I2C_BOARD_INFO("ssm2604", 0x1b), }, [--snip--] }
static int __init stamp_init(void) { [--snip--] i2c_register_board_info(0, bfin_i2c_board_info, ARRAY_SIZE(bfin_i2c_board_info)); [--snip--] return 0; } arch_initcall(board_init);
ASoC DAPM Widgets
Name | Description | Model |
---|---|---|
LOUT | Line Output for Left Channel | SSM2602, SSM2603, SSM2604 |
ROUT | Line Output for Right Channel | SSM2602, SSM2603, SSM2604 |
LLINEIN | Line Input for Left Channel | SSM2602, SSM2603, SSM2604 |
RLINEIN | Line Input for Right Channel | SSM2602, SSM2603, SSM2604 |
LHPOUT | Headphone Output for Left Channel | SSM2602, SSM2603 |
RHPOUT | Headphone Output for Right Channel | SSM2602, SSM2603 |
MICIN | Microphone Input Signal | SSM2602, SSM2603 |
ALSA Controls
Name | Description | Model |
---|---|---|
Capture Volume | Line Input PGA Volume | SSM2602, SSM2603, SSM2604 |
Capture Switch | Mute/Unmute Line Input signal | SSM2602, SSM2603, SSM2604 |
ADC High Pass Filter Switch | Enable/Disable ADC high-pass filter | SSM2602, SSM2603, SSM2604 |
Store DC Offset Switch | Store dc offset when high-pass filter is disabled | SSM2602, SSM2603, SSM2604 |
Playback De-emphasis | Select playback de-emphasis. Possible values: “None”, “32Khz”, “44.1Khz”, “48Khz” | SSM2602, SSM2603, SSM2604 |
Master Playback Volume | Headphone volume | SSM2602, SSM2603 |
Master Playback ZC Switch | Enable/Disable zero cross detection for the playback volume | SSM2602, SSM2603 |
Sidetone Playback Volume | Microphone sidetone gain control | SSM2602, SSM2603 |
Mic Boost (+20dB) | Primary microphone amplifier gain booster control. | SSM2602, SSM2603 |
Mic Boost2 (+20dB) | Additional microphone amplifier gain booster control. | SSM2602, SSM2603 |
Mic Switch | Mute/Unmute the Microphone signal | SSM2602, SSM2603 |
Output Mixer Line Bypass Switch | Mix Line Input signal into the output signal | SSM2602, SSM2603, SSM2604 |
Output Mixer HiFi Playback Switch | Mix DAC signal into the output signal | SSM2602, SSM2603, SSM2604 |
Output Mixer Mic Sidetone Switch | Mix Microphone signal into the output signal | SSM2602, SSM2603 |
Input Select | Select the ADC input signal. Possible values: “Line”, “Mic” | SSM2602, SSM2603 |
DAI configuration
The codec driver registers one DAI named “ssm2602-hifi”.
Supported DAI formats
Name | Supported by driver | Description |
---|---|---|
SND_SOC_DAIFMT_I2S | yes | I2S mode |
SND_SOC_DAIFMT_RIGHT_J | yes | Right Justified mode |
SND_SOC_DAIFMT_LEFT_J | yes | Left Justified mode |
SND_SOC_DAIFMT_DSP_A | yes | data MSB after FRM LRC |
SND_SOC_DAIFMT_DSP_B | yes | data MSB during FRM LRC |
SND_SOC_DAIFMT_AC97 | no | AC97 mode |
SND_SOC_DAIFMT_PDM | no | Pulse density modulation |
SND_SOC_DAIFMT_NB_NF | yes | Normal bit- and frameclock |
SND_SOC_DAIFMT_NB_IF | yes | Normal bitclock, inverted frameclock |
SND_SOC_DAIFMT_IB_NF | yes | Inverted frameclock, normal bitclock |
SND_SOC_DAIFMT_IB_IF | yes | Inverted bit- and frameclock |
SND_SOC_DAIFMT_CBM_CFM | yes | Codec bit- and frameclock master |
SND_SOC_DAIFMT_CBS_CFM | no | Codec bitclock slave, frameclock master |
SND_SOC_DAIFMT_CBM_CFS | no | Codec bitclock master, frameclock slave |
SND_SOC_DAIFMT_CBS_CFS | yes | Codec bit- and frameclock slave |
Supported SYSCLK rates
The codecs system clock can be configured for various input rates. When configuring the codec system clock use SSM2602_SYSCLK for the clock id.
The following list contains the supported system clock rates and their resulting sample-rates.
SYSCLK | Supported sample-rates |
---|---|
11289600 | 8kHz, 44.1kHz 88.2kHz |
12000000 | 8kHz, 32kHz, 44.1kHz 48kHz, 88.2kHz, 96kHz |
12288000 | 8kHz, 32kHz, 48kHz, 96kHz |
16934400 | 8kHz, 44.1kHz, 88.2kHz |
18432000 | 8kHz, 32kHz, 48kHz, 96kHz |
Example DAI configuration
static struct snd_soc_card bf5xx_ssm2602; static int bf5xx_ssm2602_hw_params(struct snd_pcm_substream *substream, struct snd_pcm_hw_params *params) { struct snd_soc_pcm_runtime *rtd = substream->private_data; struct snd_soc_dai *codec_dai = rtd->codec_dai; struct snd_soc_dai *cpu_dai = rtd->cpu_dai; int ret; ret = snd_soc_dai_set_fmt(cpu_dai, SND_SOC_DAIFMT_I2S | SND_SOC_DAIFMT_NB_NF | SND_SOC_DAIFMT_CBM_CFM); if (ret < 0) return ret; ret = snd_soc_dai_set_fmt(codec_dai, SND_SOC_DAIFMT_I2S | SND_SOC_DAIFMT_NB_NF | SND_SOC_DAIFMT_CBM_CFM); if (ret < 0) return ret; ret = snd_soc_dai_set_sysclk(codec_dai, SSM2602_SYSCLK, 12000000, SND_SOC_CLOCK_IN); if (ret < 0) return ret; return 0; } static struct snd_soc_ops bf5xx_ssm2602_ops = { .hw_params = bf5xx_ssm2602_hw_params, }; static struct snd_soc_dai_link bf5xx_ssm2602_dai_link[] = { { .name = "ssm2602", .stream_name = "SSM2602", .cpu_dai_name = "bfin-i2s.0", .codec_dai_name = "ssm2602-hifi", .platform_name = "bfin-i2s-pcm-audio", .codec_name = "ssm2602.0-001b", .ops = &bf5xx_ssm2602_ops, }, };
SSM2604 evaluation board driver
Source
Status
As a Module
To add support for the built-in codec SSM2602 of BF52xC to the kernel build system, a few things must be enabled properly for things to work. The configuration is as following:
Linux Kernel Configuration Device Drivers --->Sound card support ---> Advanced Linux Sound Architecture ---> < > Sequencer support OSS Mixer API OSS PCM (digital audio) API ALSA for SoC audio support ---> SoC I2S Audio for the ADI BF5xx chip SoC SSM2602 Audio support for BF52x ezkit < > SoC AC97 Audio support for BF5xx (0) Set a SPORT for Sound chip
I2C bus is used to configure the codec. So, if the audio driver is built into kernel, the I2c driver is also built into kernel automatically. But if the audio driver is built as module, then make sure that the I2C driver is loaded before the audio module.
Linux Kernel Configuration Device Drivers ---> <*> I2C support ---> --- I2C support I2C Hardware Bus Support ---> <*> Blackfin TWI I2C support
Doing this will create modules (outside the kernel). The modules will be inserted automatically when it is needed. You can also build sound driver into kernel.
Testing the built in kernel driver
If audio is configured as modules, skip this section. If audio is built into kernel and you have booted the kernel, there are a few things to check to ensure audio is working:
- Check the boot messages to see if you have booted the correct kernel. During kernel boot, it should print out:
Advanced Linux Sound Architecture Driver Version 1.0.12rc1 (Thu Jun 22 13:55:50 2006 UTC). ASoC version 0.13.1 dma rx:3 tx:4, err irq:15, regs:ffc00800 ssm2602 Audio Codec 0.1<6>dma_alloc_init: dma_page @ 0x03011000 - 512 pages at 0x03e00000 asoc: SSM2602 <-> bf5xx-i2s-0 mapping ok ALSA device list: #0: bf5xx_ssm2602 (SSM2602)
Testing the audio module
root:~> modprobe snd-ssm2602 root:~> modprobe snd-pcm-oss root:~> lsmod Module Size Used by snd_pcm_oss 31968 0 snd_mixer_oss 11360 1 snd_pcm_oss snd_ssm2602 1412 0 snd_soc_ssm2602 8528 1 snd_ssm2602 snd_soc_bf5xx 2784 1 snd_ssm2602 snd_soc_bf5xx_i2s 10916 2 snd_ssm2602,snd_soc_bf5xx snd_soc_core 17120 3 snd_ssm2602,snd_soc_ssm2602,snd_soc_bf5xx snd_pcm 48356 3 snd_pcm_oss,snd_soc_bf5xx,snd_soc_core snd_page_alloc 4232 1 snd_pcm snd_timer 13796 1 snd_pcm snd 31092 6 snd_pcm_oss,snd_mixer_oss,snd_soc_ssm2602,snd_soc_core,snd_pcm,snd_timer soundcore 3940 1 snd root:~> tone TONE: generating sine wave at 1000 Hz...
Testing Audio
- Check the output
root:~> tone TONE: generating sine wave at 1000 Hz...
You should hear something out of the headphone Jack on the top of J8. - Set the audio mixer to Mic (the default is Line, assuming you have built ALSA utils):
root:/> amixer sset 'Input Mux' 'Mic' Simple mixer control 'Input Mux',0 Capabilities: enum Items: 'Line' 'Mic' Item0: 'Mic'
Also you can run “alsamixer” to get graphic configuration interface, OSS-based “mixer” can work too. - Check to make sure mp3s work (assuming you have built mp3play),
- The first step is to download a mp3 file onto the platform. The
wget
command assumes that networking is properly configured (you have an IP number, the default gateway is set, and DNS servers can be accessed), and working.root:/> cd /var root:/var> wget http://www.radiocrazy.com/shows/A/AbbottCostello/ABCOWhosOnFirstclip.mp3
- Next, play it with mp3play:
root:/var> mp3play ABCOWhosOnFirstclip.mp3
- You can play it in one step with:
root:~> mp3play http://www.radiocrazy.com/shows/A/AbbottCostello/ABCOWhosOnFirstclip.mp3 http://www.radiocrazy.com/shows/A/AbbottCostello/ABCOWhosOnFirstclip.mp3: MPEG2-III (0 ms)
- Optionally check to make sure the Line and headphone are working properly:
root:/> amixer sset 'Input Mux' 'Line' Simple mixer control 'Input Mux',0 Capabilities: enum Items: 'Line' 'Mic' Item0: 'Line' root:~> arecord -d 10 test.wav Recording WAVE "test.wav" : Unsigned 8 bit, Rate 8000 Hz, Mono root:~> aplay test.wav
This should record 10 seconds of whatever is on the Line, and then play it back over the output. - You should also be able to do a “talkthrough”, and hear on the speakers anything you put on the line.
root:~> arecord | aplay
- 555声音发生器I2C控制
- AD5790 IDAC Linux漂流器(Wiki站点)
- AD5504 IO高体积ADC Linux漂流器
- AD1836声音Linux漂流器
- AD1845:并联端口16位声音端口立体声编码器数据Sheet
- AD1819:AC‘97声音端口编码器数据Sheet
- AD1881年:AC‘97声音Max Codec过时的数据Sheet
- AD7298 IIO多通道ADC Linux漂流器
- ADAU1361声音编解码器Linux驱动程序
- ADAU1977声音Linux漂流器
- ADAU第1373声音编解码器Linux驱动程序
- ADAU1781声音编解码器Linux驱动程序
- ADAU1701声音音频系统Linux驱动器
- SM2518声音Linux漂流器
- ADIN1200/ADIN1300 Linux漂流器
- SM401声控集成块构成的声控闪光电路 3628次阅读
- 制作一个全景声音放大器的电路原理和资料简介 3818次阅读
- 拾音器工作原理_拾音器的作用 2.3w次阅读
- 拾音器是干什么用的_拾音器和麦克风的区别 5.4w次阅读
- 拾音器怎么安装_拾音器怎么用 3.3w次阅读
- 3D环绕立体声具有哪些应用特点 7667次阅读
- 分音器如何操作 电子分音器有什么用 6468次阅读
- 音箱中的分音器的作用是什么 5609次阅读
- 声音传感器有哪些_声音传感器的应用 8.4w次阅读
- 一种监控拾音器电路的分析与制作 2.5w次阅读
- 拾音器是什么_有什么用_拾音器工作原理介绍 1.7w次阅读
- 拾音器底噪声音大怎么解决及安装注意事项 2.3w次阅读
- 拾音器和话筒分别有什么优势 2.2w次阅读
- 声音传感器模块使用说明 2.3w次阅读
- 声音传感器有哪些 1.3w次阅读
下载排行
本周
- 1电子电路原理第七版PDF电子教材免费下载
- 0.00 MB | 1491次下载 | 免费
- 2单片机典型实例介绍
- 18.19 MB | 95次下载 | 1 积分
- 3S7-200PLC编程实例详细资料
- 1.17 MB | 27次下载 | 1 积分
- 4笔记本电脑主板的元件识别和讲解说明
- 4.28 MB | 18次下载 | 4 积分
- 5开关电源原理及各功能电路详解
- 0.38 MB | 11次下载 | 免费
- 6100W短波放大电路图
- 0.05 MB | 4次下载 | 3 积分
- 7基于单片机和 SG3525的程控开关电源设计
- 0.23 MB | 4次下载 | 免费
- 8基于AT89C2051/4051单片机编程器的实验
- 0.11 MB | 4次下载 | 免费
本月
- 1OrCAD10.5下载OrCAD10.5中文版软件
- 0.00 MB | 234313次下载 | 免费
- 2PADS 9.0 2009最新版 -下载
- 0.00 MB | 66304次下载 | 免费
- 3protel99下载protel99软件下载(中文版)
- 0.00 MB | 51209次下载 | 免费
- 4LabView 8.0 专业版下载 (3CD完整版)
- 0.00 MB | 51043次下载 | 免费
- 5555集成电路应用800例(新编版)
- 0.00 MB | 33562次下载 | 免费
- 6接口电路图大全
- 未知 | 30320次下载 | 免费
- 7Multisim 10下载Multisim 10 中文版
- 0.00 MB | 28588次下载 | 免费
- 8开关电源设计实例指南
- 未知 | 21539次下载 | 免费
总榜
- 1matlab软件下载入口
- 未知 | 935053次下载 | 免费
- 2protel99se软件下载(可英文版转中文版)
- 78.1 MB | 537793次下载 | 免费
- 3MATLAB 7.1 下载 (含软件介绍)
- 未知 | 420026次下载 | 免费
- 4OrCAD10.5下载OrCAD10.5中文版软件
- 0.00 MB | 234313次下载 | 免费
- 5Altium DXP2002下载入口
- 未知 | 233046次下载 | 免费
- 6电路仿真软件multisim 10.0免费下载
- 340992 | 191183次下载 | 免费
- 7十天学会AVR单片机与C语言视频教程 下载
- 158M | 183277次下载 | 免费
- 8proe5.0野火版下载(中文版免费下载)
- 未知 | 138039次下载 | 免费
评论